Top 30 Python Interview Questions | 2022

Here we bring you some top questions that can be asked during the Python interview. If you are preparing for an interview in 2021, then it is a great chance that you might be get selected if you read all these questions and Answers.
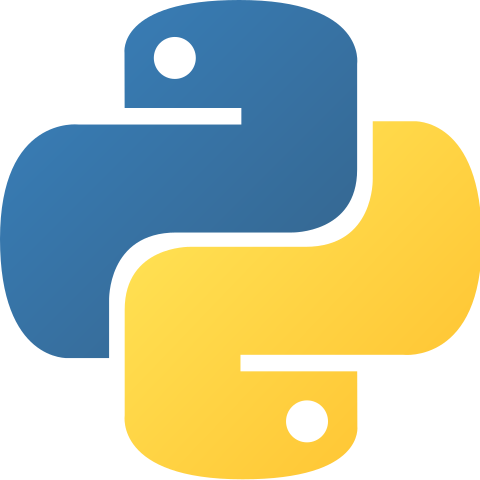
1. What do you mean by Python?
Answer: Python is a general-purpose and high-level programming language that was designed by Guido Van Rossum ( a Dutch programmer known as the best author of the Python language) in 1991. It has an object-oriented strategy that aims to help programmers write clear and logical codes for various projects. It is generally used in software development, System Scripting, Mathematics, Web development, etc.
2. What is PEP 8 in Python?
Answer: PEP 8 is described as a document that assists us in providing guidance on how to write Python code. Guido van Rossum, Barry Warsaw, and Nick Coghlan published it in 2001.
It stands for the Python Enhancement Proposal, and its main task is to improve Python code readability and reliability.
3. Explain Dynamic typed Language?
Answer: We must think about what typing is before we know what a dynamically typed language is. Typing refers to programming language type checking. “1” + 2 would result in a type error in a strongly typed language such as Python, as these languages do not permit “type-coercion” (implicit conversion of data types). On the other hand, a language that is weakly typed, like Javascript, will be simple.
Type verification can be done in two stages
Static– Data types are checked prior to execution.
Dynamic– Data types during execution are tested.
Since Python is an interpreted language, each statement line by line is executed and therefore type-checking is done on the fly during execution. Therefore, Python is a language that is dynamically typed.
4. Explain the Scope in Python?
Answer: Every Python object works within a Scope. A scope is a block of code that remains relevant to an object in Python. Namespaces define all objects within a program in a unique way. Such namespaces, however, also have a fixed scope for them to use their objects without any prefixes. The following are a few examples of the context generated in Python during code execution:
- A local Scope is defined as local objects present in the current function
- A module-level Scope is defined as global objects of current function accessible in the program.
- A global Scope is defined as the objects that are accessible since the execution of this program.
- An Outermost scope is defined as the objects that are searched last to find the name referenced. And it includes all the built-in names that are callable in the program
5. What do you mean by [::-1] in Python?
Answer: [: -1 ] is used to reverse the array or sequence order.
For instance:
import array as arr
My_Array=arr.array('i',[1,2,3,4,5])
print(My_Array[::-1])
Output: array(‘i’, [5, 4, 3, 2, 1])
6. Define the negative indexes and why are they used.
Answer: The Python sequences are indexed and consist of both positive and negative numbers. The positive numbers include ‘ 0 ‘ which is used as the first index and ‘ 1 ‘ as the second index, and this is how the process goes on.
The negative number index ends with ‘-1‘ being the last index in the sequence and ‘-2’ as the penultimate index and the series carries forward as the positive number.
The negative index is also used to denote the index to be represented in the right order.
7. Differentiate between xrange and range in Python?
Answer: The functionality of xrange() and range() is quite different. both generate an integers string, with the only difference being that range returns a Python array, while xrange() returns an xrange() object.
So, does it make any difference? It definitely does, since xrange() does not produce a static list unlike range(), it generates the value on the go. Typically, this technique is used with an object type generator known as ‘Yielding’.
In systems where memory is a constraint, yielding is necessary. In such conditions, constructing a static list as in range() can result in a Memory Error, while xrange() can handle it optimally by using just enough generator memory (significantly less compared to the generator).
xrange() can be illustrated with python2 and not with python3.
Let’s look at an example:
for i in xrange(10): # numbers from o to 9
print (i) # output => 0 1 2 3 4 5 6 7 8 9
for i in xrange(1,10): # numbers from 1 to 9
print (i) # output => 1 2 3 4 5 6 7 8 9
for i in xrange(1, 10, 2): # skip by two for next
print (i) # output => 1 3 5 7 9
8. Define the use of the break statement?
Answer: We will understand this with the help of a flow chart
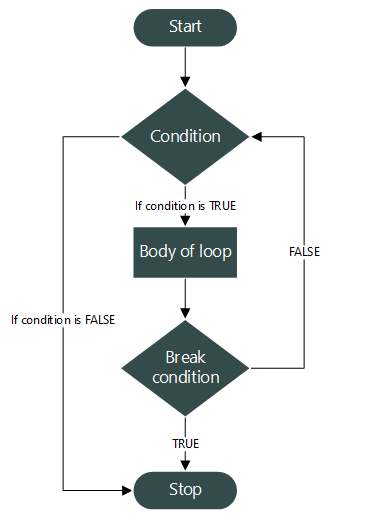
even = [2,4,6,8,10,11,12,14]
odd = 0
for val in even:
if val%2!=0:
odd = val
break
print(val)
print("Odd value found ",odd)
Output:
2
4
6
8
10
The odd value found at 11
9. What is the use of Split in Python?
Answer: This is used to separate a given string in Python.
For example :
a= “Codersera python”
print(a.spilt())
OUTPUT : [‘Codersera’, ‘python’]
10. What is Encapsulation?
Answer: Encapsulation means that the code and the data are bound together. A Python class is an example of encapsulation.
11. Define data abstraction?
Answer: Data Abstraction contains only the necessary details and hides the world’s implementation. It can be attained by using interfaces and abstract classes in Python too.
12. Explain Python decorator?
Answer: Decorators are very powerful and useful tools in Python that allow programmers to change the behavior of any class or function. It allows us to wrap up another function in order to extend the behavior of the wrapped function without permanently modifying it.
Basically, a decorator takes in a function, adds some functionality, and returns it.
Example
def make_pretty(func):
def inner():
print("I got decorated")
func()
return inner
def ordinary():
print("I am ordinary")
13. Define namespaces in Python?
Answer: A Namespace is defined as a system that is used to control the names in a program. This avoids duplication and leads to the uniqueness of names in a program.
A namespace is a basic idea for structuring and organizing software that is more useful in large projects. However, if you are new to programming, it could be a bit difficult concept to grasp. Therefore, we tried to make namespaces a little easier to understand.
14. Define Slicing in Python?
Answer: Slicing is a method used to pick a range of items such as an array, tuple, and string from the sequence type. Using the slice way, it’s helpful and simple to get elements from a set. It requires a: (colon) separating the field index start and end.
All the List or tuple forms of data collection allow us to use slicing to collect items. While we can obtain elements by defining an index, we only obtain a single element, whereas we can obtain a collection of elements by slicing.
15. What is PHYTHONPATH?
Answer: PYTHONPATH is a variable environment that can be configured to add additional directories where Python is looking for modules and packages. This is especially useful in keeping Python libraries that you don’t want to download in the default global place.
16. Define docstring in Python?
Answer: A documentation string or docstring is a multiline string that is used to document a particular segment of code.
The docstring must explain the work of method and function.
17. What does len() do?
Answer: ‘len()’ is used to measure the length of a string, an array a list, etc.
For example:
stg='ABCD'
len(stg)
18. Name the built-in types of Python?
Answer:
- Boolean
- Built-in function
- Integers
- Floating point
- Strings
- Complex numbers
Read: Best Python IDEs and Code editors
19. What do you mean by ‘pass’ in Python?
Answer: Pass defines an operation-free Python expression. In a complex phrase, it’s a placeholder. This code allows transferring the control without error if we want to create an empty class or function.
For example:
mutex = True
if (mutex == True) :
pass
else :
print("False")
20. Define the use of the help() and dir() functions?
Answer: The functions Help() and dir() are accessible from the Python interpreter and are used to view a compiled function dump.
Help() function: The help() function is used to view the documentation string and also helps us to see the file, keyword, and attribute-related support.
Dir() function: to show the specified symbols, the dir() function is used.
21. What do you mean by Iterators in Python?
Answer: In Python, Iterators, like a list, is used to iterate a set of elements. An iterator is a process of collection of objects, and that can be a list, a tuple, or a dictionary. To iterate the stored elements, Python iterator implements _itr_ and the next() method. We normally use loops in Python to iterate over the collections (list, tuple).
22. How you can create a Unicode string in Python?
Answer: In Python 3, the old Unicode type has been replaced by the “str” type, and the string is treated as Unicode by default. We can make a string in Unicode by using the art. title. encode (“utf-8”) function.
23. Define the Functions in python?
Answer: A Function is a block of code that is executed only when it is called. The def keyword is used to define the Python function.
For example
def Newfunc():
print("Hi, Welcome to Codersera”)
Newfunc(); #calling the function
Output: Hi, Welcome to Codersera.
24. Differentiate between pickling and unpickling in Python?
Answer: The pickle module accepts and transforms any Python object into a string representation and dumps it into a file using the dump function, this process is called pickling. While the method of retrieving original Python objects from the stored string representation is called unpickling.
25. Define generators?
Answer: Generators are functions that return an iterable array of items.
26. How to Capitalize the first letter of a string?
Answer: The capitalize() method in Python capitalizes a string’s first character. If at the end of the string is already a capital letter, then it returns the initial string.
27. Differentiate between Python 2.0 and Python 3.0?
Answer: Python 2. x is Python’s older version. The new and updated version of Python 3. x. Python 2. x is now heritage. Python 3. x is the language’s present and future.
The Print statement (function) is the most visible difference between Python2 and Python3. It looks like “Hello” in Python 2, and it’s written in Python 3 (“Hello”).
String in Python2 is ASCII implicitly, and in Python3 it is Unicode.
28. What is the output of [‘!!Welcome!!’]*2?
Answer: The output would be [‘!!Welcome!! ‘, ‘!!Welcome!!’]
29. How to write comments in Python?
Answer: Comments start with a # character in Python. Additionally, however, comments are sometimes made using docstrings (strings enclosed in triple quotes).
#Comments in Python start like this
print("Comments in Python start with a #")
Output: Comments in Python start with a #
30. How will you import modules in Python?
Answer: Modules would be imported with the import keywords. Let us look at the coding
import array #importing using the original module name
import array as arr # importing using an alias name
from array import * #imports everything present in the array module
Read about this also: Git Interview Questions for Developers
Wrapping Up
Heading for an interview is like walking with hundreds of questions in your head. Those questions might be what would be asked, will I be able to answer all of them correctly, etc. So, we hope that this entire list of Python questions and answers might solve your doubts if any. In case of any queries, our Codersera team of developers is here for you to help.
FAQ's
What is the most unique factor about the Python language?
Python is a high-level, interpreted language that does not require a compiler, unlike other programming languages such as Java and C++. Python code is stored in a . pyc file, which serves as the Python engine and eliminates the need for a compiler.
What is the scope of Python?
Python is a versatile programming language that can be used in a variety of fields, such as software development, government administration, business, science, arts, education, and others. According to Naukri.com, there were more than 75,000 open jobs for Python developers in India at the end of 2019.
How can we use python in app development?
Mobile app development with python helps in creating music and other types of audio and video applications. Since the internet is loaded with audio and video content, you can use Python for analyzing it all. Some Python libraries like PyDub and OpenCV also help in the successful completion of the app development.